WebVTT Cuemarker
<p class="entry-intro">
<a href="http://dev.w3.org/html5/webvtt/">WebVTT</a> is a text format
that can be used to provide captions and subtitles for HTML video.
While working on a project that uses it I found myself needing a
way to find and mark precise cue in/out times.
<a href="https://github.com/tylergaw/webvtt-cuemarker">webvtt-cuemarker</a>
is a small tool I whipped up in JavaScript to do just that.
</p>
<h2>A WebVTT Primer</h2>
<p>
The format is still fairly new and not yet widely supported, so I'll give
a quick example of how it's used.
</p>
<pre><code class="language-clike">WEBVTT FILE
00:00:01.265 --> 00:00:06.210 This is text that will appear over the video at during the in/out points.
00:00:05.500 --> 00:00:10.250 You can use HTML within WebVTT, pretty cool.
That's an example of a very simple WebVTT file with two cues. We'll
refer to it as captions.vtt
The next thing to do is to let your HTML video know about the WebVTT file.
That's done using the track
element.
<video controls>
<source src="/path/to/video.mp4" type="video/mp4">
<source src="/path/to/video.webm" type="video/webm">
<track kind="captions" label="English captions"
src="/path/to/captions.vtt" srclang="en" default></track>
</video>
That's all there is to it. If we played the fictional video above
we would see the two captions appear at the times provided in captions.vtt
.
For a more in-depth look at the track
element I suggest
Getting Started With the Track Element
on HTML5 Rocks. That's where I first learned about all this goodness.
Why Is Cuemarker Needed?
WebVTT is very specific about the time format for cues. Cue times must
be represented exactly as shown in captions.vtt
. It's
easy to type out the times in that way, but how do you find
precise times using HTML video? HTML video controls display the time
of the video, but only down to the second. And not in the format needed
for WebVTT. This is why I wrote Cuemarker.
Cuemarker provides a keyboard shortcut–the period key–for setting in/out points and outputting the cue time in the required format.
Cuemarker also provides shortcuts to interact with HTML video. You can play/pause using the space bar and seek forward/backward using the right/left arrow keys. Those controls allow you to arrive at more precise moments in video than you can using the default video scrubber provided by the browser.
How Do I Use It?
I'll go back to the HTML in the previous example, but I'm going to
remove the track
element. For marking cue times you only
need an HTML video.
<video controls>
<source src="/path/to/video.mp4" type="video/mp4">
<source src="/path/to/video.webm" type="video/webm">
</video>
Once your HTML is in place, you'll need a bit of JavaScript.
Cuemarker creates a variable–cuemarker
–in the global scope.
cuemarker
is a function that takes one required parameter;
the video element. It also takes a second, optional parameter to
specify a seek interval and output function.
cuemarker(document.querySelector('video'));
That's a bare-bones example and may be all that you'll need. The cue
times will be output using console.log
.
Here's another example using both the available options.
cuemarker(document.querySelector('video'), {
seekInterval: 0.5 //default is 0.03,
output: function (cuetime) {
var times = document.getElementById('cuetimes'),
li = document.createElement('li');
li.innerHTML = cuetime;
times.appendChild(li);
}
});
In that example, the seek interval is less precise than the default
and the cue times will be injected into the page as li
elements that are children of the ul
with an id of "cuetimes".
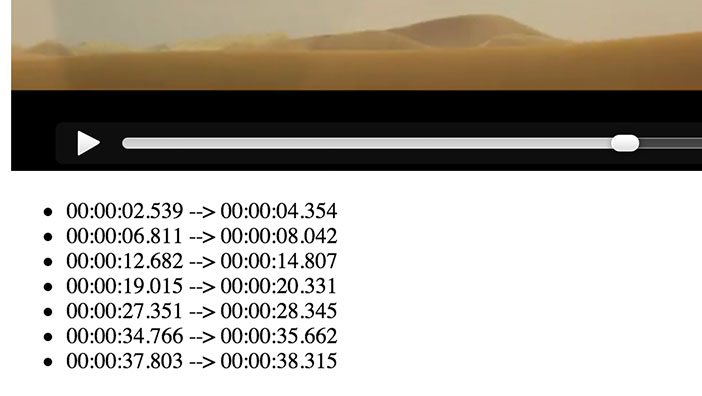
Cuemarker doesn't try to get cute by attempting to transfer cue times to a WebVTT file. All it's interested in doing is outputting the in/out times. Once you've marked in/out times you can copy them over to your WebVTT files. That's how I've been using it and it's working really well for me.
A working demo of the latter example is located here.
That's It?
Yep, that's what I got for now. Again, the track
element
and WebVTT are not yet widely supported. As of this writing only the
latest Chrome and Opera are cool enough to do so. The Web moves fast though and
I know it won't be long before Firefox and Safari follow suit.
Limited support for the underlying technology means that this is a tool you may not need today, but you might tomorrow. Fork it!
Thanks for reading